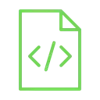
ليست هناك حاجة للنظر من النافذة أو التحقق من هاتفك باستمرار للحصول على آخر تحديثات الطقس، يمكنك إنشاء محطة الطقس الخاصة بك باستخدام الاردوينو وحساس الحرارة والرطوبة .
في هذا المشروع سنستخدم حساس DHT11 لمراقبة مستويات درجة الحرارة والرطوبة وحساس كشف المطر لمعرفة ما اذا كان هناك مطر. وأخيرًا سنعرض كل هذه البيانات على شاشة كريستالية . يمكنك استخدام نفس المشروع فى الصوبات الزراعية الذكية للتاكد من أن بيئة الصوبة مناسبة لنمو نبات معين .
. قم بتوصيل الاسلاك بين الاردوينو وحساس الحركة والشاشة الكريستالية كما هو ظاهر فى الصورة التى فى الاسفل
التوصيلات من الاردوينو الى لوحة التجارب :
• منفذ ال 5 فولت ← المنافذ الموجبة بلوحة التجارب
• منفذ الجراوند ← المنافذ السالبة بلوحة التجارب
التوصيلات من حساس الحرارة والرطوبة DHT11 :
• المنفذ الموجب الحساس الحرارة والرطوبة ← المنافذ الموجبة بلوحة التجارب
• المنفذ السالب الحساس الحرارة والرطوبة ← المنافذ السالبة بلوحة التجارب
• منفذ الاشارة الحساس الحرارة والرطوبة ← منفذ رقم 2 فى لوحة الاردوينو
التوصيلات من حساس كشف قطرات المطر :
• المنفذ الموجب لحساس كشف قطرات المطر ← المنافذ الموجبة بلوحة التجارب
• المنفذ السالب لحساس كشف قطرات المطر ← المنافذ السالبة بلوحة التجارب
• منفذ A0 لحساس كشف قطرات المطر ← منفذ رقم A0 فى لوحة الاردوينو
التوصيلات من الشاشة الكريستالية :
• المنفذ الموجب للشاشة الكريستالية ← المنافذ الموجبة بلوحة التجارب
• المنفذ السالب للشاشة الكريستالية ← المنافذ السالبة بلوحة التجارب
• المنفذ SCL للشاشة الكريستالية ← منفذ رقم A5 فى لوحة الاردوينو
• المنفذ SDA للشاشة الكريستالية ← منفذ رقم A4 فى لوحة الاردوينو
فى البداية ستعرض الشاشة الكريستالية عبارة “Voltaat Learn” لمدة 3 ثوانٍ. ومن ثم ستعرض “مرحبًا بكم في محطة الطقس” لمدة 3 ثوانٍ أخرى. ثم ستعرض قيمة درجة الحرارة بالدرجات المئوية وقيمة الرطوبة بالنسب المئوية ، إلى جانب حالة المطر.